Advanced JavaScript
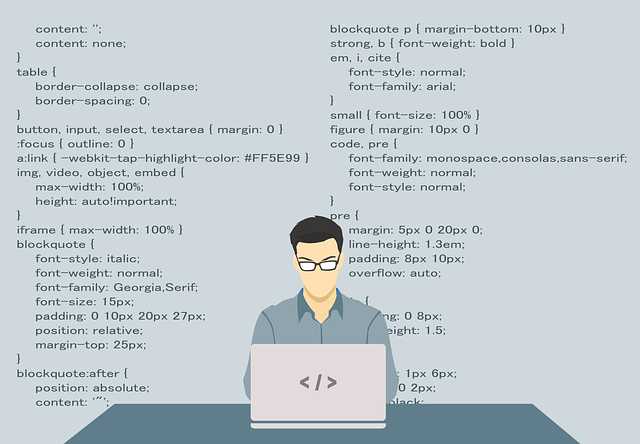
---------------------------------------------------------------------
Course Description
Advanced Javascript online course get started with core concepts of javascript. Advanced Javascript online course intention is to provide a demonstration the core mechanics of the javascript programming language.
Advanced Javascript online course will allow the participants to learn the benefits of implementing core concepts and optimization of javascript.
Advanced Javascript online course is ideally developed for programmers, developers who wants to expertise in writing code using javascript.
In the Advanced Javascript online training course, Uplatz provides an in-depth online training for the participants or learners to gain knowledge in improving the core details of javascript compilation, optimization and execution. Uplatz provides appropriate teaching and expertise training to equip the participants for implementing the learnt concepts in an enterprise.
Advanced Javascript online training course curriculum covers introduction, javascript resources, javascript compiler and object orienting.
With the help of Advanced Javascript online course, the learners can:
-
Inhouse Terminology and concepts related to the Advanced Javascript
-
Understand the key concepts of Advanced Javascript
-
Able to follow set of rules in Advanced Javascript
-
Able to execute modular and reusable code
Uplatz provides an in-depth training to the learners to accelerate their knowledge and skill set required for a Javascript Programmer.
Advanced JavaScript
Lesson 1: Introduction to Advanced JavaScript
· Welcome to Advanced JavaScript
· Accessing the Console
· Using the Console
· Good Programming Style Practices
· Testing Code in the Console
· Interacting Directly in the Console
· Commenting Your Code
Lesson 2: Know Your Types
· Know Your Types: Primitives and Objects
· Primitives
· Some Interesting Numbers
· Objects
· Enumerating Object Properties
· Primitives That Act like Objects
· JavaScript is Dynamically Typed
Lesson 3: Truthy, Falsey, and Equality
· Truthy, Falsey, and Equality
· Values That are Truthy or Falsey
· Implied Typecasting
· Testing Equality
· Objects and Truthy-ness
· Objects and Equality
Lesson 4: Constructing Objects
· Constructing JavaScript Objects
· Constructing an Object with a Constructor Function
· Constructing an Object Using a Literal
· Constructing an Object Using a Generic Object Constructor
· So, What's the Best Way to Make an Object?
· Initializing Values in Constructors
· this
· Constructing Array Objects
Lesson 5: Prototypes and Inheritance
· Object-Oriented Programming in JavaScript
· instanceof
· Prototypes
· Prototypes of Literal Objects
· What is a Prototype Good For?
· The Prototype Chain
· Prototypal Inheritance
· When are Prototype Objects Created?
· hasOwnProperty
· __proto__
· Setting the Prototype Property to an Object Yourself
Lesson 6: Functions
· JavaScript Functions
· What is a Function?
· Different Ways of Defining a Function
· Functions as First Class Values
· Anonymous Functions
· Returning a Function from a Function
· Functions as Callbacks
· Calling Functions: Pass-by-Value
· Return
Lesson 7: Scope
· Scope
· Variable Scope
· Function Scope
· Hoisting
· Nested Functions
· Lexical Scoping
· Scope Chains
· Inspecting the Scope Chain
Lesson 8: Invoking Functions
· Invoking Functions
· Different Ways to Invoke Functions
· What Happens to this When You Invoke a Function
· Nested Functions
· When You Want to Control How this is Defined
· call() and apply()
· Function Arguments
· The Four Ways to Invoke a Function
Lesson 9: Invocation Patterns
· Invocation Patterns
· Recursion
· Why Use Recursion?
· Chaining (a la jQuery)
· Static vs. Instance Methods
Lesson 10: Encapsulation and APIs
· Encapsulation and APIs
· Privacy, Please
· An Example
· Private Variables
· Private Functions
· A Public Method
· Accessing a Public Method from a Private Function
· Encapsulation and APIs
Lesson 11: Closures
· Closures
· Making a Closure
· What is a Closure?
· Playing with Closures
· Each Closure is Unique
· Closures Might Not Always Act Like You Expect
· Closures for Methods
· Using Closures
· Where We've Used Closures Before
Lesson 12: The Module Pattern
· Module Pattern
· IIFE or Immediately Invoked Function Expressions
· The Module Pattern
· Using the Module Pattern with JavaScript Libraries
· A Shopping Basket Using the Module Pattern
· Why Not Just Use an Object Constructor?
Lesson 13: The JavaScript Environment
· JavaScript Runs in an Environment
· The Core Language, and the Environment's Extensions
· How the Browser Runs JavaScript Code
· Including JavaScript in Your Page
· The JavaScript Event Loop
· The Event Queue
· Asynchronous Programming
· JavaScript in Environments Other Than the Browser
Lesson 14: ECMAScript 5.1
· The ECMAScript Standard for JavaScript
· Strict Mode
· New Methods
· Object Property Descriptors
· Sealing and Freezing Objects
· Creating Objects
------------------------------------------------------------------------------------------------------------
Advanced Javascript online certification course with the help of expert professionals training is recognized across the globe. Because of the increased adoption of the Advanced Javascript in various companies the participants are able to find the job opportunity easily. The leading companies hire Javascript Programmer considering their skill of understanding concepts of advanced javascript. Advanced Javascript online certification course is known for their knowledge in developing interactive websites. After pursuing Advanced Javascript online certification course the participants can become as a web developer, Javascript coder, front-end web developer, back-end web developer and can pursue a wide range of career paths.
Advanced JavaScript Interview Questions & Answers
-------------------------------------------------------------------------------------------------------
1) Explain what is Javascript?
Javascript is an object-oriented computer programming language commonly used to create interactive effects within web browsers.It is first used by the Netscape browser, that provides access to the HTML document object model (DOM), provides access to the browser object model (BOM). Javascript syntax looks a lot like java, c or c++ syntax.
Below is the list of data types supported by Javascript:-
- Undefined
- Null
- Boolean
- String
- Symbol
- Number
- Object
2) What close() does in Javascript?
In Javascript close() method is used to close the current window. You must write window.close() to ensure that this command is associated with a window object and not some other JavaScript object.
3) What is the difference between let and var?
Both var and let are used for variable/ method declaration in javascript but the main difference between let and var is that var is function scoped whereas let is block scoped.
4) Is it possible to write a multi-line string in JavaScript?
Yes, JavaScript provides the facility to write a String in multiple lines
5) Discuss a way to convert the string of any base to integer in JavaScript?
We can convert a string of any base to the integer if we wish with the help of the JavaScript built-in method called parseInt(). This method has two parameters: string and radix. The second parameter is optional and used when you want to get the integer equivalent to the radix as the base. The default value is 10 for decimals values.
6) Explain Closures in JavaScript?
Closures are the combination of lexical environment and function within which the function was declared. This allows JavaScript programmers to write better, more creative, concise and expressive codes. The closure will consist of all the local variables that were in-scope when the closure was created.
Sure, closures appear to be complex and beyond the scope, but after you read this article, closures will be much more easy to understand and more simple for your everyday JavaScript programming tasks. JavaScript is a very function-oriented language it gives the user freedom to use functions as the wish of the programmer.
7) Explain JavaScript Event Delegation Model?
In JavaScript, there is some cool stuff that makes it the best of all. One of them is Delegation Model. When capturing and bubbling, allow functions to implement one single handler to many elements at one particular time then that is called event delegation. Event delegation allows you to add event listeners to one parent instead of specified nodes. That particular listener analyzes bubbled events to find a match on the child elements. Many people think it to be complicated but in reality, it is very simple if one starts understanding it.
8) Describe negative infinity in JavaScript?
NEGATIVE_INFINITY property represents negative infinity and is a number in javascript, which is derived by ‘dividing negative number by zero’. It can be better understood as a number that is lower than any other number. Its properties are as follows:
– A number of objects need not to be created to access this static property.
– The value of negative infinity is the same as the negative value of the infinity property of the global object.
9) Explain function hoisting in JavaScript?
JavaScript’s default behavior that allows moving declarations to the top is called Hoisting. The 2 ways of creating functions in JavaScript are Function Declaration and Function Expression.
10) What is the use of let & const in JavaScript?
In modern javascript let & const are different ways of creating variables. Earlier in javascript, we use the var keyword for creating variables. let & const keyword is introduced in version ES6 with the vision of creating two different types of variables in javascript one is immutable and other is mutable.
const: It is used to create an immutable variable. Immutable variables are variables whose value is never changed in the complete life cycle of the program.
let: let is used to create a mutable variable. Mutable variables are normal variables like var that can be changed any number of time.
11) When should I use Arrow functions in ES6?
- Use function in the global scope and for Object.prototype properties.
- Use class for object constructors.
- Use => everywhere else.
12) What are the possible ways to write a function isInteger(value), that determines if the value is an integer?
EcmaScript 6 introduced a new function, Number.isInteger(), to determine whether a value is an integer or not. But the specifications before EcmaScript 6 have no such function. In fact, there are no integers; numeric values are always in the form of floating-point values.
13) Explain Arrow functions?
An arrow function is a consise and short way to write function expressions in Es6 or above.A rrow functions cannot be used as constructors and also does not supports this, arguments, super, or new.target keywords. It is best suited for non-method functions.
14) What are exports and imports?
Imports and exports help us to write modular javascript code. Using Imports and exports we can split our code into multiple files. Imports allow taking only some specific variables or methods of a file. We can import methods or variables that are exported by a module. See the below example for more detail.
15) What is difference between module.exports and export?
The module is a plain JavaScript object with an exports property. Exports is a plain JavaScript variable that happens to be set to module.exports. At the end of your file, node.js will basically ‘return’ module.exports to the require function. A simplified way to view a JS file in Node could be this:
var module = { exports: {} };
var exports = module.exports;
// your code
return module.exports;
If you set a property on exports, like exports.a = 9;, that will set module.exports.a as well because objects are passed around as references in JavaScript, which means that if you set multiple variables to the same object, they are all the same object; so then exports and module.exports are the same objects.
But if you set exports to something new, it will no longer be set to module.exports, so exports and module.exports are no longer the same objects.
16) How to import all exports of a file as an object?
import * as object name from ‘./file.js’ is used to import all exported members as an object. You can simply access the exported variables or methods using dot (.) operator of the object.
Example:
objectname.member1;
objectname.member2;
objectname.memberfunc();
17) Explain “use strict”?
“use strict” is a javascript directive that is introduced in Es5. The purpose of using “use strict” directive is to enforce the code is executed in strict mode. In strict mode we can’t use a variable without declaring it. “use strict” is ignored by earlier versions of Javascript.
18) Explain Event bubbling and Event Capturing in JavaScript?
Event Capture and Bubbling: In HTML DOM API there are two ways of event propagation and determines the order in which event will be received. The two ways are Event Bubbling and Event Capturing. The first method event bubbling directs the event to its intended target, and the second is called event capture in which the event goes down to the element.
19) In Javascript are calculations with fractional numbers guaranteed to be precise?
NO, calculations with fractional numbers are not guaranteed to be precise in Javascript
20) List the comparison operators supported by Javascript?
Javascript supports below comparison operators
- > Greater than
- < Less than
- <= Less than or equal to
- >= Greater than or equal to
- == Equal to
- != Not Equal to
- === Equal to with datatype check
- !== Not equal to with datatype check
21) How do you declare variables in Javascript?
In Javascript, variables are declared using the var keyword.A variable must begin with A letter, $ or _.
eg. var myVar=”Online Interview Questions”;
PS: All variables in Javascript are Case sensitive.
22) What will happen if an infinite while loop is run in Javascript?
The program will crash the browser.
23) How to get the last index of a string in Javascript?
string.length-1 is used to get the last index of a string in Javascript
Example Usage:-
var myString="JavascriptQuestions";
console.log(myString.length-1);
24) How to get the primitive value of a string in Javascript?
In Javascript valueOf() method is used to get the primitive value of a string.
Example Usage:
var myVar= "Hi!"
console.log(myVar.valueOf())
25) What are the primitive data types in JavaScript?
A primitive is a basic data type that’s not built out of other data types. It can only represent one single value. All primitives are built-in data types by necessity, (the compiler has to know about them,) but not all built-in data types are primitives.
In JavaScript there are 5 primitive data types are available they are undefined, null, boolean, string and number are available.Everything else in Javascript is an object.
26) What does the instanceof operator do?
In Javascript instanceof operator checks whether the object is an instance of a class or not:
Example Usage
Square.prototype = new Square();
console.log(sq instanceof Square); // true
27) What is Javascript BOM?
BOM stands for “Browser Object Modal” that allows Javascript to ‘talk’ to the browser, no standards, modern browsers implement similar BOMS – window, screen, location, history, navigator, timing, cookies.
28) What are different types of Popup boxes available in Javascript?
In Javascript there are 3 types of Popup Boxes are available, they are
- Alert
- Confirm
- Prompt
29) How can you create an array in Javascript?
There are 3 different ways to create an array in Javascript. They are
- By array literal
usage:
var myArray=[value1,value2...valueN];
- By creating instance of Array
usage:
var myArray=new Array();
- By using an Array constructor
usage:
var myArray=new Array('value1','value2',...,'valueN');
30) What is the 'Strict' mode in JavaScript and how can it be enabled?
Strict mode is a way to introduce better error-checking into your code. When you use strict mode, you cannot, for example, use implicitly declared variables, or assign a value to a read-only property, or add a property to an object that is not extensible.
You can enable strict mode by adding “use strict”; at the beginning of a file, a program, or a function. This kind of declaration is known as a directive prologue. The scope of a strict mode declaration depends on its context. If it is declared in a global context (outside the scope of a function), all the code in the program is in strict mode. If it is declared in a function, all the code in the function is in strict mode.
31) How to calculate Fibonacci numbers in JavaScript?
Calculating Fibonacci series in JavaScript
Fibonacci numbers are a sequence of numbers where each value is the sum of the previous two, starting with 0 and 1. The first few values are 0, 1, 1, 2, 3, 5, 8, 13 ,…,
function fib(n) {
var a=0, b=1;
for (var i=0; i < n; i++) {
var temp = a+b;
a = b;
b = temp;
}
return a;
}
32) What is the difference between the substr() and substring() functions in JavaScript?
Difference between the substr() and substring() functions in JavaScript.
The substr() function has the form substr(startIndex,length). It returns the substring from startIndex and returns ‘length’ number of characters.
var s = "hello";
( s.substr(1,4) == "ello" ) // true
The substring() function has the form substring(startIndex,endIndex). It returns the substring from startIndex up to endIndex – 1.
var s = "hello";
( s.substring(1,4) == "ell" ) // true
33) What are different types of Inheritence? Which Inheritance is followed in JavaScript.
There are two types of Inherientence in OOPS Classic and Prototypical Inheritance. Javascript follows Prototypical Inheritance.
34) What is output of undefined * 2 in JavaScript?
nan is output of undefined * 2.
35) How to add/remove properties to object dynamically in JavaScript?
You can add a property to an object using object.property_name =value, delete object.property_name is used to delete a property.
36) How to convert Javascript date to ISO standard?
toISOString() method is used to convert javascript date to ISO standard. It converts JavaScript Date object into a string, using the ISO standard.
Usage:
var date = new Date();
var n = date.toISOString();
console.log(n);
// YYYY-MM-DDTHH:mm:ss.sssZ
37) How to get inner Html of an element in JavaScript?
InnerHTML property of HTML DOM is used to get inner Html of an element in JavaScript.
38) How to clone an object in JavaScript?
Object.assign() method is used for cloning an object in Javascript.Here is sample usage
var x = {myProp: "value"};
var y = Object.assign({}, x);
39) Explain Typecasting in JavaScript?
In Programming whenever we need to convert a variable from one data type to another Typecasting is used. In Javascript, we can do this via library functions. There are basically 3 typecasts are available in Javascript Programming, they are:
- Boolean(value): Casts the inputted value to a Boolean
- Number(value): Casts the inputted value to an Integer or Floating point Number.
- String(value) : Casts the inputted value value a string
40) How to encode and decode a URL in JavaScript?
encodeURI() function is used to encode an URL in Javascript.It takes a url string as parameter and return encoded string. Note: encodeURI() did not encode characters like / ? : @ & = + $ #, if you have to encode these characters too please use encodeURIComponent(). Usage:
var uri = "my profile.php?name=sammer&occupation=pāntiNG";
var encoded_uri = encodeURI(uri);
decodeURI() function is used to decode an URL in Javascript.It takes a encoded url string as parameter and return decoded string. Usage:
var uri = "my profile.php?name=sammer&occupation=pāntiNG";