Java Programming Basics
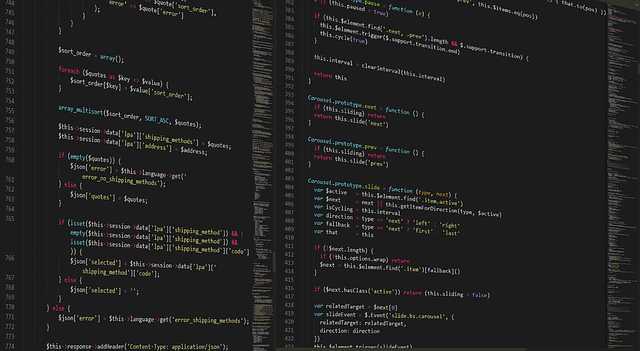
§ Object Oriented
-----------------------------------------------------------------------------------------------------------------------------
Java Programming Basics
Java Programming Fundamentals course syllabus
Java Overview
· How the Java Environment Works
· HelloWorld Program
· Launch Single-File Source Code
· jShell REPL
· Comments and Terminators
· Identifiers
Syntax and Types
· Java Variables
· var declarations
· Java Types
· Basic Java Types
· Reference types
· Conditional Expressions
· Logical operators
· If statement
· While Statement
· Do Statement
· For statement
· Special flow of control operators
· Switch statement
· Numerical Operators
· Casting
· Strings
Classes and Objects
· What is an Object?
· Objects and Encapsulation
· What is a Class?
· Class Object Relationship
· The Person class
· Variable types
· Constructors
· Working with the class Person
Working with Methods
· Method Definitions
· Method Arguments & Return Types
· Overloading Methods
· Method Invocations
· Defining methods
· Constructors Versus Methods
· toString() method
· Adding behaviour to the class Person
· The PersonApp program
Class Inheritance
· Inheritance in Java
· Implementing Inheritance
· Rule for overriding methods
· Rules for Polymorphic variables
· Casting and Inheritance
· The super variable
· Constructors and Inheritance
Abstract Classes, Class Side Behaviour and Final
· Abstract Classes
· Abstract Classes in Java
· Defining an Abstract Class
· Extending an Abstract Class
· Using Concrete Subclasses
· Class Side Information
· Class Side Data
· Class Side Behaviour
· Final Keyword
Java Interfaces and Enumerations
· What is an Interface in Java?
· Basic Interface Definitions
· Implementing an Interface
· Interface
· Using an Interface in a Contract
· Inheritance by Interfaces and Types
· Classes and multiple Interfaces
· Default Interface Methods
· Static Interface Methods
· Enumeration Support
· Implementing Enumerated Types
Packages
· Packages
· Class-Package Relationship
· Declaring Packages
· Packaging the Person class
· Role of the Classpath
· JAR Files
· Encapsulation and Packages
· Class modifier
· Constructor Modifier
· Variable Modifier
· Method Modifiers
· Package Summary
Java 9+ Modules
· Introduction the JPMS
· Java Platform Module System
· Why we need modules
· Look at what modules are
· How to define a module
· How to create a single module application
· How to link modules
Arrays
· What is a Java Array?
· Creating arrays of Objects
· Accessing Array Elements
· Main method args array
· Short hand from
· Ragged Arrays in Java
· Working with Ragged Arrays
· Implications of Inheritance for Arrays
· Integer Array Example
Java Generics
-
Generics and Basic Types
-
Generics and Their Types
-
Adding Generics to your classes
-
A simple user defined Generic class (the Bag class)
-
Type Equality
-
Generic Collection Assignment
-
Generics and Inheritance
Collections Classes
-
Collections API
-
ArrayList
-
Interfaces v Concrete Classes
-
HashMap
-
Iteration and Enumeration
-
Queues
-
Generics and Collections
-
For Loops
-
Boxing and Unboxing
-
Raw Collections
Java 9+ Immutable Collections
-
Why Immutability?
-
Immutability
-
List.of Factory method
-
Set.of Factory method
-
Map.of Factory method
-
Immutable Collection Nodes
-
Java 10 Enhancement
Error and Exception Handling
-
Errors & Exceptions
-
Exception types in Java
-
Part of the Exception Hierarchy
-
Exception Handling
-
Local Handling
-
Exception Handling Example
-
Passing the Buck
-
Try with Resources
-
Defining new Exceptions
-
Chained Exceptions
Nested / Inner Classes
-
Four types of Nested / Inner Class
-
Properties of Member level inner classes
-
Properties of Method Inner classes
-
Anonymous Method Inner classes
-
Java 11 Nest-based Access
Java Functions
-
Functional Programming
-
Functional Programming in Java
-
Functional Interfaces
-
Using Functional Interfaces
-
Lambdas in Java
-
Closing / Capturing Variables
-
Returning Functions
-
Combining Functions
-
Higher Order Functions
-
Defining Lambdas
-
Method References
Java Optional Type
-
Null considered Harmful
-
Java Optional Type
-
Optional Variables
-
Creating Optional values
-
Method Summary
Java 9 Streams
-
Streams
-
Streams from Collections
-
Terminal / Non Terminal Operators
-
Creating a Stream
-
Map Operation
-
Collectors
-
Filter operation
-
Sorted operation
-
ForEach
-
Pipelining Operations
-
Parallel Streams
-
Not just collections
Files, Paths and IO Streams
-
Introducing NIO.2
-
Paths class and Path Interface
-
The Files class
-
File Attributes
-
The File Watcher Service
-
IO Streams
-
Scanners
-----------------------------------------------------------------------------------------------------------------------------
Java Developer
Software Developer