Perl Programming
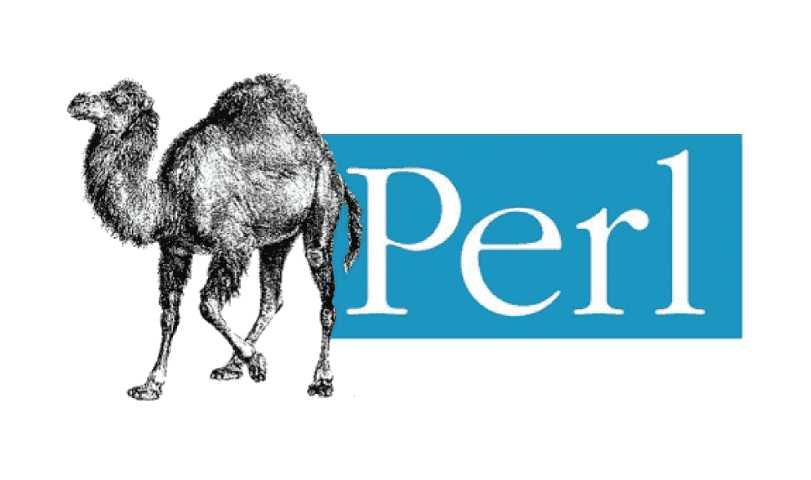
Perl Programming Course Overview
This is a Perl Programming course by Uplatz.
The Perl Programming course is designed to give delegates the knowledge to develop/maintain Perl scripts.
The delegate will learn and acquire skills as follows:
- Declare variables and initialise them
- Understand scalar and list context
- Construct expressions with arithmetic, logical and relational operators
- Use iterative type statements
- Use conditional statements
- Read/write text and binary files
- Manipulate text using regular expression
- Use and code subroutines
- Use built-in library functions
- Use arrays and hashes
- Declare and use packages
Who will the Course Benefit?
Programmers who need to write or maintain scripts in Perl.
--------------------------------------------------------------------------------------------------------------------------------------------
Course Objectives
Knowledge of Perl to write, maintain and understand Perl scripts.
--------------------------------------------------------------------------------------------------------------------------------------------
Perl Programming
Perl Programming Training Course
Course Introduction
- Administration and Course Materials
- Course Structure and Agenda
- Delegate and Trainer Introductions
Session 1: INTRODUCTION TO PERL
- What is PERL and why PERL is useful
- Obtaining PERL
Session 2: PERL BASICS
- Using Perl
- Comments in a Perl Script
- Data Representation in Perl
- Numbers
- Strings
- Comparators
- Operators
- Using Standard Input
- Standard Output
Session 3: ARRAYS AND LISTS
- What is a List or Array?
- Assignment
- Accessing Array Elements
- The Push and Pop Functions
- The Shift and Unshift Functions
- Building an Array from ‹STDIN›
Session 4: CONDITIONAL AND CONTROL STRUCTURES
- Control Structures
- Statement Blocks
- Conditional Statements
- If Keyword
- Unless Keyword
- Elsif Keyword
- Control Statements
- While Keyword
- Until Keyword
- Do Keyword
- For Statement
- Foreach Statement
Session 5: ASSOCIATIVE ARRAYS
- Associative (Hash) Arrays
- Keys Function
- Values Function
- Each Function
- Delete Function
- Exist Function
- Big Arrow
Perl Programming Training Course
Session 6: BASIC INPUT AND OUTPUT
- STDIN
- The Diamond Operator
- Print for Normal Output
- Printf for Formatted Output
Session 7: REGULAR EXPRESSION
- Regular Expression
- Multipliers
- Special Perl Variables in Pattern Matching
- Substitutions
- The Split and Join Functions
- Split Function
- Join Function
- Case Statement
Session 8: FUNCTIONS
- A User Defined Function (Subroutine)
- Calling a User Function
- Return Values
- Passing Arguments to Functions
- Private Variables in Functions
- Variables Using Local
Session 9: ADDITIONAL CONTROL STRUCTURES
- Additional Control Structures
- Last Statement
- Next Statement
- Redo Statement
- Labeled Blocks
- Expression Modifiers
- && and || as Control Structures
Perl Programming Training Course
Session 10: FILE HANDLING
- Filehandles and File Tests
- Opening and Closing a Filehandle
- Using Filehandles
- File Tests
Session 11: PERL DBI/DBD MODULES
- Perl DBI Module
- What is DBI
- Database Handles
- Statement Handles
Session 12: FILE AND DIRECTORY FUNCTIONS
- File and Directory Functions
- Changing Directories
- Current Directory
- Globbing
- Opening and Closing a Directory
- Reading a Directory Handle
- Removing a File
- Renaming a File
- Making and Removing Directories
- Modifying Permissions
- Modifying Ownership
Session 13: MODULES PACKAGES LIBRARIES PRAGMA & PERLDOC
- Modules
- Package
- Library
- Pragma
- Perldoc
------------------------------------------------------------------------------------------------------------------
Perl Programming Interview Questions
------------------------------------------------------------------------------------------------------------------1) Explain what is Perl language?
Perl stands for “Practical Extraction and Reporting Language”. It’s a powerful scripting language and is rich in features. Using Perl, we can write powerful and efficient code that can be used in mission-critical projects.
2) What are the various advantages and disadvantages of Perl?
Advantages of Perl include:
- Perl is efficient and is easy-to-use.
- It is an Interpreted language i.e. Perl program is interpreted on a statement-by-statement basis.
- Perl is portable and cross-platform. Currently, it can run on more than 100 platforms.
- Perl is extendable. We can include various open-source packages and modules in a Perl program for any additional functionality. For example, we can import CPAN modules for database support in the Perl program.
The main disadvantage of Perl is that as it is an interpreted language, the execution speed is quite slow. Although it allows us to write high-level code, we cannot write complex code using Perl. Perl has too many features that can be exhaustive for a programmer to comprehend.
3) What are the various uses of Perl?
Perl is used in a mission-critical project – like the defense industry. It is also used in “Rapid Prototyping”.
4) Explain the various characteristics of Perl.
Enlisted below are the various Characteristics of Perl:
- Case-sensitive
- Easy to code
- Open-source
- Portable and cross-platform.
- Extendable
- No distinction between the types of variables.
- Can return non-linear types likes arrays etc.
- Non-scalars can be used as loop indices.
- Supports high-level intrinsic operations – E.g. stack Push/pop.
- Powerful text manipulation API including Regular expressions.
5) Explain the execution of a program in Perl.
Perl is portable and Perl programs can be executed on any platform. Though having a Perl IDE is useful, we can even write the Perl code in a notepad and then execute the program using the command prompt.
6) What are the various flags/arguments that can be used while executing a Perl program?
The following arguments can be used while executing a Perl program.
- w – argument shows a warning.
- d – used for debugging.
- c – compiles only do not run.
- e – execute.
We can also use a combination of arguments like:
pl –wd filename.pl
Variables In Perl
7) Comment on data types and variables in Perl.
Perl variables do not have a data type. The data type of a variable in Perl is inferred from its value.
Value needs to be assigned to a variable before using it. Without this, the program may result in an unexpected output.
8) What are Scalars in Perl?
Variables having values with linear data types like integer, float or string are called scalar variables in Perl.
$x=10;
$mystr=”abc”;
These are all scalar variables.
9) Comment on the scope of variables in Perl.
By default, all the variables in Perl are global in scope. This means that a variable can be used for a reminder of the program from the point of its declaration.
You can use ‘my’ keyword for a variable and this makes a variable to have local scope.
Example: my $x=10;
10) What are Numeric Operators in Perl?
Numeric operators in Perl are as follows:
- Arithmetic operators (+,-,*/).
- Comparison operators to compare two numbers (>, <, ==, !=,<=,>=,<=>).
- Bitwise Operators(&(and), |(or),^(ex-or),~(not),<<(shift left),>>(shift right)).
Arithmetic operators perform from left to right while Bitwise operators perform an operation from right to left.
11) Why do you create an Application for Real Time System in which processing speed is vital?
Perl is used in the following cases:
• To process large text
• When data manipulation is done by application
• If you require fast developments expand to become libraries
• To load database operations
12) How can you represent the warning signs in the Perl in case of an error and what are the options through which this task can be performed?
There is an option in Perl which is known as WCommand Line. All the warning messages can be displayed using this and the pragma function simply makes sure that the user can declare the variables during appearance of warning messages. The entire program can be scrolled easily and in fact, in a very short span of time using the in-built debugger.
13) Distinguish My and Local?
The variables which are declared using “my” lives only in that particular block ion which they are declared and inherited functions do not have a visibility that are called in that block. The variables which are defined as “local” are visible in that block and they have a visibility in functions which are called in that particular block.
14) Differentiate Use and Require?
Use:
- This method is used for modules.
- The objects which are included are varied at compilation time.
- You need not give a file extension.
Require:
- This method is used for both modules and libraries.
- The objects are included are verified at run time.
- You need not give file extension.
15) While writing a program, why the code should be as short as possible?
Complex codes are not always easy to handle. They are not even easy to be reused. Moreover, finding a bug in them is not at all a difficult job. Any software or application if have complex or lengthy code couldn’t work smoothly with the hardware and often have compatibility issues. Generally, they take more time to operate and thus becomes useless or of no preference for most of the users. The short code always makes sure that the project can be made user-friendly and it enables programmers to save a lot of time.
16) Why Perl Patterns are not Regular Expressions?
Perl patterns have back references
By the definition, a regular expression should determine next state infinite automation without extra money to keep in previous state. State machine is required by the pattern / ([ab] +) c1/ to remember old states. Such patterns are disqualified as being regular expressions in the term’s classic sense.
17) What happens if a Reference is returned to Private Variable?
Your variables are kept on track by the Perl, whether dynamic or else, and does not free things before you use them.
18) Why -w Argument is used with Perl Programs?
-w option of the interpreter is used by most of the Perl developers especially in the development stage of an application. It is warning option to turn on multiple warning messages that are useful in understanding and debugging the application.
19) Which has highest precedence in between List and Terms? Explain.
In Perl, the highest precedence is for Perl. Quotes, variables, expressions in parenthesis are included in the Terms. The same level of precedence as Terms is for List operators. Especially, these operators have strong left word precedence.
20) Define a Short Circuit Operator?
The C-style operator ll carries out logical operation which is used to tie logical clauses, overall value of true is returned if either clause is true. This operator is known as short-circuit operator because you need not check or evaluate right operand if the left operand is true.
21) In Perl, name different forms of Goto and Explain?
In Perl, there are three different forms for goto, they are:
• goto name
• goto label
• goto expr
goto name is used along with subroutines, it is used only when it is required as it creates destruction in programs. It is the second form of label where Execution is transferred to a statement labeled LABEL using goto LABEL. The last label form is goto EXPR which expects EXPR to evaluate label.
22) Can you add two arrays together?
Yes, it is possible to add two arrays together with a push function. A value or values to end of the array is added using push function. The values of list are pushed on to the end of an array using push function. Length of list is used to increase length of an array.
23) How Shift Command is used?
The first value of an array shifted using shift array function and it is returned, which results in array shortening by one element and moves everything from a place to left. If an array is not specified to shift, shift uses @ ARGV, the command line arguments of an array is passed to script or to an array named @.
24) Explain different types of Eval Statements?
In general, there are two types of eval statements they are:
• Eval BLOCK and
• Eval EXPR
An expression is executed by eval EXPR and BLOCK is executed by eval BLOCK. Entire block is executed by eval block, BLOCK. When you want your code passed in expression then first one is used and to parse code in the block, second one is used.
25) Describe returning values from Subroutines?
The value of last expression which is evaluated is the return value of subroutine or explicitly, a returned statement can be used to exit subroutine which specifies return value. This return value is evaluated in perfect content based on content of subroutine call.
26) What are the two different types of Data Perl Handles?
Perl handles two types of data they are
(i) Scalar Variables and
(ii) Lists
Scalar variables hold a single data item whereas lists hold multiple data items.
27) What are Scalar Variables?
Scalar variables are what many programming languages refer to as simple variables. They hold a single data item, a number, a string, or a perl reference. Scalars are called scalars to differentiate them from constructs that can hold more than one item, like arrays.
28) Explain about Lists?
A list is a construct that associates data elements together and you can specify a list by enclosing those elements in parenthesis and separating them with commas. They could themselves be arrays, hashes or even other lists. Lists do not have a specific list data type.
29) Name all the Prefix Dereferencer in Perl?
The symbol that starts all scalar variables is called a prefix dereferencer. The different types of dereferencer are.
(i) $-Scalar variables
(ii) %-Hash variables
(iii) @-arrays
(iv) &-subroutines
(v) Type globs-*myvar stands for @myvar, %myvar.
30) Explain about an Ivalue?
An ivalue is an item that can serve as the target of an assignment. The term I value originally meant a “left value”, which is to say a value that appears on the left. An ivalue usually represents a data space in memory and you can store data using the ivalues name. Any variable can serve as an ivalue.
31) How does a "grep" Function perform?
Grep returns the number of lines the expression is true. Grep returns a sub list of a list for which a specific criterion is true. This function often involves pattern matching. It modifies the elements in the original list.
32) Explain about Typeglobs?
Type globs are another integral type in perl. A typeglob`s prefix derefrencer is *, which is also the wild card character because you can use typeglobs to create an alias for all types associated with a particular name. All kinds of manipulations are possible with typeglobs.
33) Is there any way to add two arrays together?
Of course you can add two arrays together by using push function. The push function adds a value or values to the end of an array. The push function pushes the values of list onto the end of the array. Length of an array can be increased by the length of list.
34) How to use the Command Shift?
Shift array function shifts off the first value of the array and returns it, thereby shortening the array by one element and moving everything from one place to the left. If you don’t specify an array to shift, shift uses @ ARGV, the array of command line arguments passed to the script or the array named @-.
35) What exactly is Grooving and Shortening of the array?
You can change the number of elements in an array simply by changing the value of the last index of/in the array $#array. In fact, if you simply refer to a nonexistent element in an array perl extends the array as needed, creating new elements. It also includes new elements in its array.
36) What are the three ways to empty an array?
The three different ways to empty an array are as follows
1) You can empty an array by setting its length to a negative number.
2) Another way of empting an array is to assign the null list ().
3) Try to clear an array by setting it to undef, but be aware when you set to undef.
37) How do you work with Array Slices?
An array slice is a section of an array that acts like a list, and you indicate what elements to put into the slice by using multiple array indexes in square brackets. By specifying the range operator you can also specify a slice.
38) What is meant by Splicing Arrays. Explain in Context of List and Scalar.
Splicing an array means adding elements from a list to that array, possibly replacing elements now in the array. In list context, the splice function returns the elements removed from the array. In scalar context, the splice function returns the last element removed.
39) What are the different types of Perl Operators?
There are four different types of perl operators they are
(i) Unary operator like the not operator
(ii) Binary operator like the addition operator
(iii) Tertiary operator like the conditional operator
(iv) List operator like the print operator
40) Which has the highest precedence, List or Terms? Explain.
Terms have the highest precedence in perl. Terms include variables, quotes, expressions in parenthesis etc. List operators have the same level of precedence as terms. Specifically, these operators have very strong left word precedence.
------------------------------------------------------------------------------------------------------------------