React
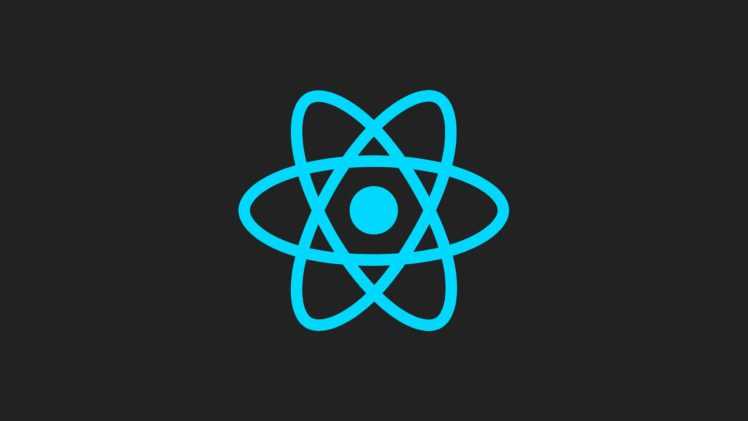
React Course Overview
The React course comprises sessions dealing with setting up for and creating a React app, JSX and element rendering, components and props, state and lifecycle, hooks, event handling, lists and keys, forms, composition and inheritance, React Router, Flux, and Redux. React Native is not covered on this course.
Exercises and examples are used throughout the course to give practical hands-on experience with the techniques covered.
-------------------------------------------------------------------------------------------------------------------------------------------
Who will the Course Benefit?
The React course is designed for JavaScript developers who are interested in using React to build fast, single page, client-side web applications.
--------------------------------------------------------------------------------------------------------------------------------------------
Course Objectives
This course aims to provide the delegate with the knowledge to be able to build a Single Page Application (SPA) composed of React components and incorporating React Router. The delegate will also be exposed to application state containers including Flux and Redux and local state management via Hooks.
----------------------------------------------------------------------------------------------------------------------------------------
This is a React course by Uplatz.
React
React Training Course
Course Introduction
- Administration and Course Materials
- Course Structure and Agenda
- Delegate and Trainer Introductions
Session 1: INTRODUCTION
- Understanding React
- Using Babel
- Create-React-App
- Setup & Project Structure
Session 2: MODERN JAVASCRIPT
- Single Page Applications
- Transpilers & Polyfills
- ES6 Features
- Primitive & Reference Types
- Template Literals
- Destructuring
- Let, Const & Var
- Arrow Functions
- Understanding Classes
- Decorators
- Spread and Rest Operators
- Default Parameters and Values
- Exports and Imports
- Modules
- Array Functions
- Promises
- Generator Functions
- Sets and Maps
- Functional JavaScript
- Typescript
Session 3: JSX & ELEMENT RENDERING
- Virtual DOM
- JSX
- Lists and Keys
Session 4: COMPONENTS & PROPS
- What is a Component
- Functional vs. Class Components
- React Props
- State in Brief
- Typechecking
- Composition & Inheritance
React Training Course
Session 5: STATE & LIFECYCLE
- State vs. Props
- Adding State
- Lifting State Up
- Component Lifecycle
- Component Lifecycle Methods
- Immutability
Session 6: EVENT HANDLING
- Handler Assignment
- Binding to this
- Passing Arguments to Event Handlers
- Custom Components & Events
- Synthetic Event
Session 7: FORMS
- Controlled Components
- Uncontrolled Components
- Using Refs
- Forms JSX Summary
- Validation
- Form Libraries
Session 8: REACT ROUTER
- Using react-router
- Core Components
React Training Course
Session 9: HOOKS
- Motivation: Stateful Logic Re-Use
- Functional Vs Class Components Refactored
- Local State Without A Class: Internals
- Standard Hooks
- Custom Hooks: The Anticipated Future
- The (Only) Rules of Hooks
Session 10: FURTHER REACT
- Testing React
- React Performance
- Production Build/Deploy
- Refs & DOM
- React Patterns
- Authentication
- JSON Web Token
- Third Party Components
React Training Course
Session 11: FLUX
- Flux vs MVC
- Flux Main Components
- Flux Flow in Action
- Flux Utils
- Flux Implementations
Session 12: INTRODUCING REDUX
- Redux: Inspired by Flux
- Core Redux: Actions, Action Creators, Reducers, Store
- Redux Data Flow
Session 13: REDUX & REACT
- Introduction and Setup Environment
- Components of React Redux
- React Data Flow
- Redux Recipes
- Redux DevTools
Session 14: FURTHER REDUX
- Middleware
- Redux Thunk
- Redux Saga
----------------------------------------------------------------------------------------------------------------------------------------
React.js Interview Questions & Answers
----------------------------------------------------------------------------------------------------------------------------------------1) Differentiate between Real DOM and Virtual DOM.
Real DOM |
Virtual DOM |
1. It updates slow. |
1. It updates faster. |
2. Can directly update HTML. |
2. Can’t directly update HTML. |
3. Creates a new DOM if element updates. |
3. Updates the JSX if element updates. |
4. DOM manipulation is very expensive. |
4. DOM manipulation is very easy. |
5. Too much of memory wastage. |
5. No memory wastage. |
2) What is React?
- React is a front-end JavaScript library developed by Facebook in 2011.
- It follows the component based approach which helps in building reusable UI components.
- It is used for developing complex and interactive web and mobile UI.
- Even though it was open-sourced only in 2015, it has one of the largest communities supporting it.
3) What are the features of React?
Major features of React are listed below:
i. It uses the virtual DOM instead of the real DOM.
ii. It uses server-side rendering.
iii. It follows uni-directional data flow or data binding.
4) List some of the major advantages of React.
Some of the major advantages of React are:
i. It increases the application’s performance
ii. It can be conveniently used on the client as well as server side
iii. Because of JSX, code’s readability increases
iv. React is easy to integrate with other frameworks like Meteor, Angular, etc
v. Using React, writing UI test cases become extremely easy
5) What are the limitations of React?
Limitations of React are listed below:
i. React is just a library, not a full-blown framework
ii. Its library is very large and takes time to understand
iii. It can be little difficult for the novice programmers to understand
iv. Coding gets complex as it uses inline templating and JSX
6) What is JSX?
JSX is a shorthand for JavaScript XML. This is a type of file used by React which utilizes the expressiveness of JavaScript along with HTML like template syntax. This makes the HTML file really easy to understand. This file makes applications robust and boosts its performance.
7) Why can’t browsers read JSX?
Browsers can only read JavaScript objects but JSX in not a regular JavaScript object. Thus to enable a browser to read JSX, first, we need to transform JSX file into a JavaScript object using JSX transformers like Babel and then pass it to the browser.
8) What do you understand from “In React, everything is a component.”
Components are the building blocks of a React application’s UI. These components split up the entire UI into small independent and reusable pieces. Then it renders each of these components independent of each other without affecting the rest of the UI.
9) Explain the purpose of render() in React.
Each React component must have a render() mandatorily. It returns a single React element which is the representation of the native DOM component. If more than one HTML element needs to be rendered, then they must be grouped together inside one enclosing tag such as
etc. This function must be kept pure i.e., it must return the same result each time it is invoked.
10) What is Props?
Props is the shorthand for Properties in React. They are read-only components which must be kept pure i.e. immutable. They are always passed down from the parent to the child components throughout the application. A child component can never send a prop back to the parent component. This help in maintaining the unidirectional data flow and are generally used to render the dynamically generated data.
11) What is a state in React and how is it used?
States are the heart of React components. States are the source of data and must be kept as simple as possible. Basically, states are the objects which determine components rendering and behavior. They are mutable unlike the props and create dynamic and interactive components. They are accessed via this.state().
12) Why we use JSX?
- It is faster than regular JavaScript because it performs optimization while translating the code to JavaScript.
- Instead of separating technologies by putting markup and logic in separate files, React uses components that contain both.
- t is type-safe, and most of the errors can be found at compilation time.
- It makes easier to create templates.
13) What do you understand by Virtual DOM?
A Virtual DOM is a lightweight JavaScript object which is an in-memory representation of real DOM. It is an intermediary step between the render function being called and the displaying of elements on the screen. It is similar to a node tree which lists the elements, their attributes, and content as objects and their properties. The render function creates a node tree of the React components and then updates this node tree in response to the mutations in the data model caused by various actions done by the user or by the system.
14) How can you embed two or more components into one?
You can embed two or more components into the following way:
import React from 'react'
class App extends React.Component {
render (){
return (
Hello World
)
}
}
class Example extends React.Component {
render (){
return (
Hello JavaTpoint
)
}
}
export default App
15) How can you update the State of a component?
We can update the State of a component using this.setState() method. This method does not always replace the State immediately. Instead, it only adds changes to the original State. It is a primary method which is used to update the user interface(UI) in response to event handlers and server responses.
16) What is arrow function in React? How is it used?
The Arrow function is the new feature of the ES6 standard. If you need to use arrow functions, it is not necessary to bind any event to 'this.' Here, the scope of 'this' is global and not limited to any calling function. So If you are using Arrow Function, there is no need to bind 'this' inside the constructor. It is also called 'fat arrow '(=>) functions.
17) What is an event in React?
An event is an action which triggers as a result of the user action or system generated event like a mouse click, loading of a web page, pressing a key, window resizes, etc. In React, the event handling system is very similar to handling events in DOM elements. The React event handling system is known as Synthetic Event, which is a cross-browser wrapper of the browser's native event.
18) What are synthetic events in React?
A synthetic event is an object which acts as a cross-browser wrapper around the browser's native event. It combines the behavior of different browser's native event into one API, including stopPropagation() and preventDefault().
19) Explain the Lists in React.
Lists are used to display data in an ordered format. In React, Lists can be created in a similar way as we create it in JavaScript. We can traverse the elements of the list using the map() function.
20) What is the significance of keys in React?
A key is a unique identifier. In React, it is used to identify which items have changed, updated, or deleted from the Lists. It is useful when we dynamically created components or when the users alter the lists. It also helps to determine which components in a collection needs to be re-rendered instead of re-rendering the entire set of components every time. It increases application performance.
21) How are forms created in React?
Forms allow the users to interact with the application as well as gather information from the users. Forms can perform many tasks such as user authentication, adding user, searching, filtering, etc. A form can contain text fields, buttons, checkbox, radio button, etc.
React offers a stateful, reactive approach to build a form. The forms in React are similar to HTML forms. But in React, the state property of the component is only updated via setState(), and a JavaScript function handles their submission. This function has full access to the data which is entered by the user into a form.
22) What are the different phases of React component's lifecycle?
The different phases of React component's lifecycle are:
Initial Phase: It is the birth phase of the React lifecycle when the component starts its journey on a way to the DOM. In this phase, a component contains the default Props and initial State. These default properties are done in the constructor of a component.
Mounting Phase: In this phase, the instance of a component is created and added into the DOM.
Updating Phase: It is the next phase of the React lifecycle. In this phase, we get new Props and change State. This phase can potentially update and re-render only when a prop or state change occurs. The main aim of this phase is to ensure that the component is displaying the latest version of itself. This phase repeats again and again.
Unmounting Phase: It is the final phase of the React lifecycle, where the component instance is destroyed and unmounted(removed) from the DOM.
-----------------------------------------------------------------------------------------------------------