Android Development
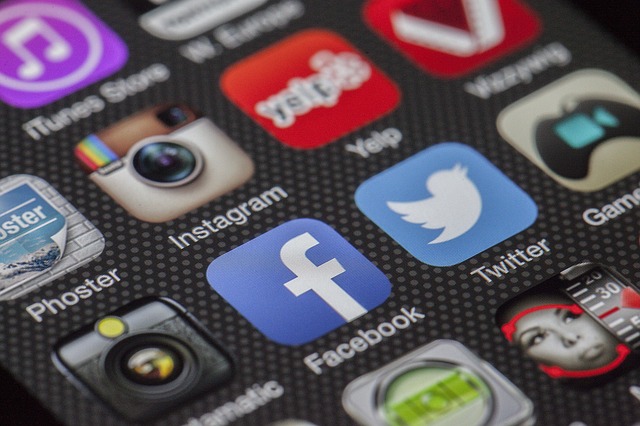
Android Development
Course Level: Beginner
This course is designed for beginners who wish to become a mobile application
developer by using Android, which is one of the most popular programming
languages for mobile application development. It is a classroom-based course
that covers the essential topics to start programming with Android.
Prerequisites:
No existing knowledge on Android is required. This course is suitable for those
who already have basic Java programming knowledge.
1. Java Topics related to Android Development
o Inheritance
o Interface
o Inner class
o Collection Framework
o Other important topics relevant to Android
2. Environment Setup for Android Studio
o Installing the Studio
o Create Android Virtual Device
3. Android Architecture & Components
o Android Runtime
o Application Framework o Activities
o Services
o Broadcast Receivers
o Content Providers
4. Hello World Example
o Create Android Application
o Structure of Android Application o The Activity File
o The Manifest File
o The Layout File
o The Strings File
o The R File
5. Resource Organizing & Accessing
o Alternative Resources
o Accessing Resources in Code o Accessing Resources in XML
6. UI Layouts
o Android Layout Types o Linear Layout
o Relative Layout
o Layout Attributes
7. UI Controls & Attributes
o TextView
o EditText
o Button
o CheckBox
o ToggleButton
o RadioGroup & RadioButton o ImageView
o Other controls
8. Style & Themes
o Defining Styles
o Using Styles
o Style Inheritance
o Android Themes
o Default Styles and Themes
9. Event Handling
o Event listeners & handlers o Event Listener Registration
§ UsinganAnonymousInnerClass
§ UsingActivityImplementsListenerInterface § Usingxmlattributeinlayout
10. Intents
o Intent Objects
o Android Intent Standard Action
o Types of Intent
o Passing and Receiving Data with Intent Extras
11. ListView and GridView
o ListView Attributes
o Array Adapter
o GridView Attributes
12. Making a ToDo List App with Login and Registration Form
o Designing an app concept
o Creating Layout with components o Creating Activities
o Implementing logic
o Building a working application
o Installing and Running on Device
13. Android Persistence
o Shared Preference
o SQLite Database
14. Android File I/O
o Internal Storage o SD card Storage
15. Google Maps API V2
o Showing current location
o Adding marker
o Customize Marker
16. Android PHP/MySQL
o PHP/MySQL basics
o Creating database & tables with data
o PHP – GET and POST Methods
o Android connecting to MySQL
17. Android working with network data
o Background process
o Connecting via GET Method
o Connecting via POST Method
o JSON Parsing
18. Custom Listview with remote data
o Async Task
o API Call
o Base Adapter
19. Android Miscellaneous Topics
o Sending Mail, SMS
o Phone Calls
o Media Player
20. Android App with all possible functionalities
o Concept discussion
o Design and wire-framing
o Layout design
o Logic development (actual coding)
o Binding of data and navigation
o Final touches
o App key store generation
o Publish