Overview Course - Java Programming
This Java course covers the length and breadth of this platform-independent and object-oriented language.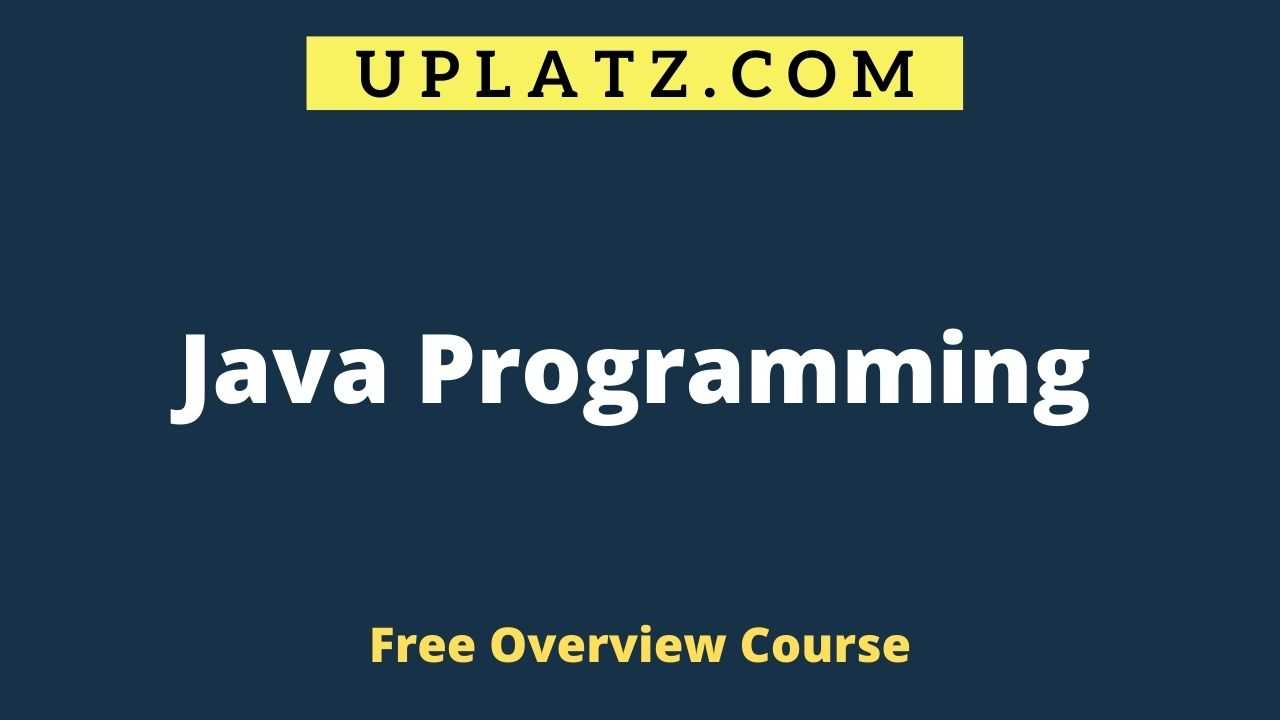
75% Started a new career BUY THIS COURSE (USD 14)
-
55% Got a pay increase and promotion
Students also bought -
-
- Java Programming Basics
- 20 Hours
- USD 41
- 2872 Learners
-
- Bundle Course - Java (Core Java - JSP - Java Servlets)
- 50 Hours
- USD 27
- 4202 Learners
-
- JavaScript Programming
- 35 hours
- USD 41
- 518 Learners
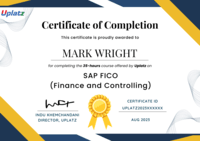
This is an overview course on Java Programming while the complete detailed-level Java Programming course is available at - https://training.uplatz.com/online-it-course.php?id=java-programming-basics-110. Java programming is one of the most widely used computer programming languages in today’s time. Be it writing a program for web applications to large systems development, Java programming is used everywhere nowadays. It is a highly reliable, fast and secured programming language and as such, Java has been one of the most favourite programming languages for the developers and other IT professionals.
Java programming environment consists of mainly 3 key components namely; (i) Java Development Kit (ii) Java Runtime Environment and (iii) Java Virtual Machine. Java has got a number of advantages which makes it a better choice for the developers. Some of them are (i) Java works on different platforms (ii) It is open-source and free to use (iii) It is a secure programming language along with being a fast and reliable platform (iv) It is Object-Oriented and as such makes it easy for the developers to reuse the code, resulting in lowering the cost.
In this Java Programming Course from Uplatz, learners will be able to get an overview of the Java Programming basics, its syntax and types, Classes and Objects in Java, Java Interfaces, Arrays and many more. Learning this course from Uplatz, will help the students in gaining a complete knowledge on the Java Programming Language by which they can upgrade their skills and land up in a high paying job in any organization.
At the end of this course, students will be awarded a Course Completion Certificate on Java Programming by Uplatz.
Course/Topic - Java Programming overview - all lectures
-
Lecture 1 - Introduction to Java
-
Lecture 2 - Data Types
-
Lecture 3 - Control Statements
• Describe how to compile and run a simple Java application
• Describe how to construct simple variables and arrays
• Describe how to construct simple expressions and control flow
• Identify object-oriented programming concepts
• Describe how Java implements object-oriented programming
• Describe the structure of classes
• Describe how simple inheritance is used
• Describe class and variable access modifiers
Java Overview
• How the Java Environment Works
• HelloWorld Program
• Launch Single-File Source Code
• jShell REPL
• Comments and Terminators
• Identifiers
Syntax and Types
• Java Variables
• var declarations
• Java Types
• Basic Java Types
• Reference types
• Conditional Expressions
• Logical operators
• If statement
• While Statement
• Do Statement
• For statement
• Special flow of control operators
• Switch statement
• Numerical Operators
• Casting
• Strings
Classes and Objects
• What is an Object?
• Objects and Encapsulation
• What is a Class?
• Class Object Relationship
• The Person class
• Variable types
• Constructors
• Working with the class Person
Working with Methods
• Method Definitions
• Method Arguments & Return Types
• Overloading Methods
• Method Invocations
• Defining methods
• Constructors Versus Methods
• toString() method
• Adding behaviour to the class Person
• The PersonApp program
Class Inheritance
• Inheritance in Java
• Implementing Inheritance
• Rule for overriding methods
• Rules for Polymorphic variables
• Casting and Inheritance
• The super variable
• Constructors and Inheritance
Abstract Classes, Class Side Behaviour and Final
• Abstract Classes
• Abstract Classes in Java
• Defining an Abstract Class
• Extending an Abstract Class
• Using Concrete Subclasses
• Class Side Information
• Class Side Data
• Class Side Behaviour
• Final Keyword
Java Interfaces and Enumerations
• What is an Interface in Java?
• Basic Interface Definitions
• Implementing an Interface
• Interface
• Using an Interface in a Contract
• Inheritance by Interfaces and Types
• Classes and multiple Interfaces
• Default Interface Methods
• Static Interface Methods
• Enumeration Support
• Implementing Enumerated Types
Packages
• Packages
• Class-Package Relationship
• Declaring Packages
• Packaging the Person class
• Role of the Classpath
• JAR Files
• Encapsulation and Packages
• Class modifier
• Constructor Modifier
• Variable Modifier
• Method Modifiers
• Package Summary
Java 9+ Modules
• Introduction the JPMS
• Java Platform Module System
• Why we need modules
• Look at what modules are
• How to define a module
• How to create a single module application
• How to link modules
Arrays
• What is a Java Array?
• Creating arrays of Objects
• Accessing Array Elements
• Main method args array
• Shorthand from
• Ragged Arrays in Java
• Working with Ragged Arrays
• Implications of Inheritance for Arrays
• Integer Array Example
Java Generics
• Generics and Basic Types
• Generics and Their Types
• Adding Generics to your classes
• A simple user-defined Generic class (the Bag class)
• Type Equality
• Generic Collection Assignment
• Generics and Inheritance
Collections Classes
• Collections API
• ArrayList
• Interfaces v Concrete Classes
• HashMap
• Iteration and Enumeration
• Queues
• Generics and Collections
• For Loops
• Boxing and Unboxing
• Raw Collections
Java 9+ Immutable Collections
• Why Immutability?
• Immutability
• List.of Factory method
• Set.of Factory method
• Map.of Factory method
• Immutable Collection Nodes
• Java 10 Enhancement
Error and Exception Handling
• Errors & Exceptions
• Exception types in Java
• Part of the Exception Hierarchy
• Exception Handling
• Local Handling
• Exception Handling Example
• Passing the Buck
• Try with Resources
• Defining new Exceptions
• Chained Exceptions
Nested / Inner Classes
• Four types of Nested / Inner Class
• Properties of Member level inner classes
• Properties of Method Inner classes
• Anonymous Method Inner classes
• Java 11 Nest-based Access
Java Functions
• Functional Programming
• Functional Programming in Java
• Functional Interfaces
• Using Functional Interfaces
• Lambdas in Java
• Closing / Capturing Variables
• Returning Functions
• Combining Functions
• Higher-Order Functions
• Defining Lambdas
• Method References
Java Optional Type
• Null considered Harmful
• Java Optional Type
• Optional Variables
• Creating Optional values
• Method Summary
Java 9 Streams
• Streams
• Streams from Collections
• Terminal / Non-Terminal Operators
• Creating a Stream
• Map Operation
• Collectors
• Filter operation
• Sorted operation
• ForEach
• Pipelining Operations
• Parallel Streams
• Not just collections
Files, Paths and IO Streams
• Introducing NIO.2
• Paths class and Path Interface
• The Files class
• File Attributes
• The File Watcher Service
• IO Streams
• Scanners
By the end of this course, you will have:
• Learnt about Java class and Objects
• Explored defining methods and properties
• Examined Class Inheritance
• Understood Java Interfaces and Enumerations
• Considered how to use Packages and Modules
• Learnt about Functional Programming in Java
• Understood how to handle Errors and Exceptions
• Worked with Files, paths and Stream IO
• Used the new Streams API for processing data
• Explored the Collection classes
The Java Programming Certification ensures you know planning, production and measurement techniques needed to stand out from the competition.
Java is a general-purpose programming language that is class-based and object-oriented. The programming language is structured in such a way that developers can write code anywhere and run it anywhere without worrying about the underlying computer architecture. It is also referred to as write once, run anywhere (WORA).
Java is based on C and C++. The first Java compiler was developed by Sun Microsystems and was written in C using some libraries from C++. Java files are converted to bit code format using a compiler that the Java interpreter then executes. Java code runs on Java Virtual Machine (JVM)—the runtime environment.
Java is a well-structured, object-oriented language, which can be considered easy for beginners. You can master it quite rapidly, as there are many processes that run automatically. You don't have to delve into “how the things work in there” too deep. Java is a cross-platform language.
Java is a well-structured, object-oriented language, which can be considered easy for beginners. You can master it quite rapidly, as there are many processes that run automatically. You don't have to delve into “how the things work in there” too deep. Java is a cross-platform language.
Uplatz online training guarantees the participants to successfully go through the Java Programming Certification provided by Uplatz. Uplatz provides appropriate teaching and expertise training to equip the participants for implementing the learnt concepts in an organization.
Course Completion Certificate will be awarded by Uplatz upon successful completion of the Java Programming online course.
The Java Programming draws an average salary of $120,000 per year depending on their knowledge and hands-on experience.
The Java SE 8 Oracle Certified Associate (OCA) certification helps you build a foundational understanding of Java, and gaining this certification credential is the first of two steps in demonstrating you have the high-level skills needed to become a professional Java developer.
The strong community, enterprise support, and growing popularity among programmers show that Java is set to stay the first choice for most businesses. Hence, java career opportunities are not fading anytime soon.
However, most jobs require a set of skills. Specialization is helpful, but technical versatility is also critical. For example, you might get a job to write Java code that connects to a MySQL database.
Note that salaries are generally higher at large companies rather than small ones. Your salary will also differ based on the market you work in.
Java Developer.
Software Development.
Software Engineer Development.