TypeScript
Unlock the full potential of modern web development by mastering TypeScript, from core syntax to full-stack integration and project deployment.
91% Started a new career BUY THIS COURSE (
USD 12 USD 41 )-
86% Got a pay increase and promotion
Students also bought -
-
- Angular: Full-Stack Application Development
- 23 Hours
- USD 12
- 725 Learners
-
- Bundle Combo - Software Testing (Manual and Automation)
- 50 Hours
- USD 23
- 555 Learners
-
- C++ Programming
- 10 Hours
- USD 12
- 463 Learners
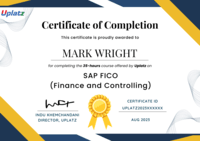
TypeScript – Self-Paced Online Course
Boost your web development skills and become a next-generation developer with this all-in-one course on TypeScript. Designed for learners at all levels, this self-paced course includes in-depth video tutorials, real-world projects, and practical exercises to help you master everything from TypeScript basics to full-stack development.
TypeScript, a statically typed superset of JavaScript, enables developers to write more reliable, readable, and scalable code. By learning TypeScript, you’ll improve your productivity and code quality, and be prepared to work on enterprise-scale applications across front-end and back-end platforms.
Whether you're a beginner transitioning from JavaScript or an experienced developer expanding your toolkit, this course delivers the practical skills needed to succeed in modern development environments using TypeScript.
By the end of this course, learners will be able to:
- Understand the fundamentals of TypeScript and its advantages over JavaScript.
- Write clean and type-safe code using advanced TypeScript features.
- Work with object-oriented programming concepts like classes, interfaces, and inheritance.
- Build modular applications with TypeScript and integrate with tools like React, Vue, Angular, and Node.js.
- Leverage utilities such as generics, decorators, namespaces, and mixins.
- Create type-safe libraries, reusable components, and scalable app architectures.
- Develop and debug TypeScript applications using tools like Jest, Babel, and Webpack.
- Build full-stack applications using Express.js and React/Redux with TypeScript.
- Implement robust state management and API integration in both web and mobile environments.
- Prepare TypeScript projects for production, testing, and deployment.
TypeScript - Course Syllabus
Module 1: Introduction to TypeScript
- Introduction to TypeScript
- What is TypeScript?
- Why should we learn TypeScript?
- TypeScript vs JavaScript
- Setting Up TypeScript Environment
- Installing TypeScript
- Writing your first TypeScript program
- Understanding the TypeScript project structure
- Configuring tsconfig.json
Module 2: TypeScript Basics
- Basic TypeScript Syntax
- Primitive types (string, number, boolean, etc.)
- Explicit vs inferred types
- Complex Types
- Arrays, Tuples, and Enums
- Union and Intersection types (with examples)
- Advanced TypeScript Types
- Interfaces and Type Aliases
- Default values, unions, and intersections
- Type Assertions, Function Types, and Generics
- Type assertions
- Function types
- Introduction to Generics
Module 3: Object-Oriented TypeScript
- Working with Classes and Objects
- Creating classes and objects
- Access modifiers (public, private, protected)
- Readonly properties
- Advanced Class Features
- Getters and setters
- Static properties and methods
- Abstract classes and inheritance
- Object-Oriented Programming in TypeScript
- Implementing OOP principles
- Hands-on project
Module 4: TypeScript Modules and Integration
- Understanding TypeScript Modules
- Organizing and using modules
- Importing and exporting
- Integrating TypeScript with JavaScript Frameworks
- Using TypeScript with React and Node.js
- Declaration Files and Frontend Tooling
- Using declaration files (.d.ts)
- Working with frontend tools like Webpack and Babel
Module 5: TypeScript Utilities and Features
- Useful TypeScript Utilities
- Utility types (Partial, Readonly, Record, etc.)
- Best practices
- Function Types and Overloading
- Function signatures
- Overloading functions
- Deep Dive into TypeScript Classes and Inheritance
- Extending classes
- Using mixins
- Exploring Access Modifiers and Readonly Properties
- Real-world examples
Module 6: Hands-on TypeScript Projects
- Building a Modular Application
- Structuring a scalable TypeScript app
- Building a Type-Safe Library
- Creating a library with strict typing
- TypeScript with Angular
- Setting up an Angular project with TypeScript
- Using TypeScript with Vue.js
- Developing a Vue.js project with TypeScript
Module 7: Error Handling, Debugging, and Compilation
- Error Handling in TypeScript
- Catching and managing errors
- Debugging TypeScript code
- Understanding TypeScript Compiler Options
- Exploring tsc options
Module 8: Advanced TypeScript Concepts
- Working with TypeScript Mixins
- Creating reusable mixins
- Building a Notification System using Mixins
- Hands-on project
- Exploring TypeScript Decorators
- Class, method, and property decorators
- Advanced Generics in TypeScript
- Conditional types and mapped types
Module 9: Full-Stack TypeScript Development
- Building a REST API with TypeScript
- Setting up an Express.js backend with TypeScript
- Creating Endpoints and Handling Requests
- CRUD operations
- Setting Up a TypeScript Frontend Project
- Configuring a frontend app
- State Management with TypeScript
- Using Vanilla TypeScript, Context API, and Redux
- TypeScript Routing
- Handling navigation with React Router
- API Integration with TypeScript
- Using Axios with TypeScript
- Unit Testing in TypeScript
- Testing with Jest
- Preparing for Production and Deployment
- Best practices for production-ready TypeScript apps
Module 10: TypeScript for Mobile Development
- Why Use TypeScript with React Native?
- Benefits of TypeScript in mobile apps
- Creating a React Native Project with TypeScript
- Step-by-step guide using Expo
Module 11: TypeScript Namespaces and Utility Projects
- Understanding TypeScript Namespaces
- Organizing code with namespaces
- Quote Generation Project with TypeScript
- Step-by-step project
- Blog Post Manager Project
- CRUD operations in TypeScript
- Blog Post Manager with SQLite
- Database integration
- Building a Password Generator in TypeScript
- Node.js and React implementations
Module 12: Interview Preparation & Conclusion
- Common TypeScript Interview Questions
- Explanation with examples and tables
Upon successful completion of the TypeScript course, learners will receive a Course Completion Certificate from Uplatz, validating their expertise in modern web development using TypeScript.
This certificate is a powerful credential to showcase your proficiency with type-safe coding practices, scalable architecture, and integration with leading frameworks like Angular, React, Vue, and Node.js.
Mastering TypeScript significantly boosts your employability in today’s development landscape, where scalable, maintainable code is essential. TypeScript is widely adopted in enterprise and startup environments across web and mobile ecosystems.
Job Roles You Can Pursue:
- TypeScript Developer
- Front-End Developer (React/Angular/Vue + TS)
- Full-Stack JavaScript Developer
- Node.js Engineer with TypeScript
- Mobile App Developer (React Native + TS)
- TypeScript Architect or Consultant
Industries Hiring TypeScript Professionals:
- Software Development & IT Services
- Finance & Banking Technology
- eCommerce & Retail
- SaaS & Cloud Platforms
- Media & Publishing
- Healthcare Technology
- What are the key differences between TypeScript and JavaScript?
TypeScript adds static typing and compile-time checks to JavaScript, enabling better tooling, error prevention, and refactoring. - How does TypeScript handle types in comparison to JavaScript?
TypeScript allows both explicit and inferred typing, enabling developers to define types for variables, functions, and objects. - What are Union and Intersection types in TypeScript?
Union types allow a variable to hold multiple types (string | number), while intersection types combine multiple types (TypeA & TypeB). - Explain interfaces vs. type aliases.
Interfaces define object shapes and support extension, while type aliases are more flexible and can represent any type including primitives and unions. - How are generics used in TypeScript?
Generics allow writing reusable and type-safe code, enabling components and functions to handle multiple data types. - What are access modifiers in classes?
Access modifiers (public, private, protected) control the visibility of class properties and methods, enforcing encapsulation. - What are declaration files in TypeScript?
Declaration files (.d.ts) provide type definitions for existing JavaScript code, enabling TypeScript to understand third-party libraries. - How does TypeScript integrate with React or Node.js?
TypeScript can be configured in React using tsx files and works seamlessly with Node.js using ts-node or compiled output. - What are decorators in TypeScript?
Decorators are annotations for classes and members that enable meta-programming, commonly used in Angular and advanced design patterns. - How can TypeScript improve debugging and code maintenance?
With static type checks, intelligent tooling, and better refactor support, TypeScript reduces runtime errors and makes code easier to manage.
- What is TypeScript used for?
TypeScript is used to develop scalable web and mobile applications with static typing, improving code quality and maintainability. - Who should take this course?
JavaScript developers, front-end and back-end engineers, and aspiring full-stack developers who want to level up their skills. - Is this course beginner-friendly?
Yes, the course starts with the basics and gradually progresses to advanced full-stack and mobile development using TypeScript. - What frameworks are covered in the course?
The course covers integration with React, Angular, Vue.js, Node.js, and React Native. - Will I receive a certificate after completing the course?
Yes, a professional course completion certificate will be issued by Uplatz. - Is there any hands-on project work included?
Yes, learners will build modular apps, REST APIs, reusable libraries, and mobile projects. - Does this course cover debugging and testing?
Absolutely. The course includes error handling, debugging, and unit testing using tools like Jest and the TypeScript compiler. - Can I use TypeScript in mobile app development?
Yes. The course includes hands-on experience with React Native and Expo using TypeScript. - Will I learn how to deploy a TypeScript application?
Yes, deployment and production-ready optimization techniques are covered in detail. - Is this course suitable for full-stack development?
Yes, you’ll learn to build end-to-end applications using TypeScript on both frontend and backend.