Java Fundamentals: The Java Language
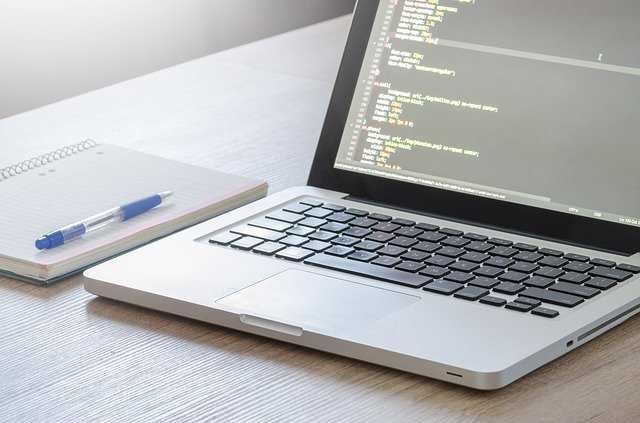
Take your first step towards a career in software development with this introduction to Java—one of the most in-demand programming languages and the foundation of the Android operating system. Designed for beginners, this Specialization will teach you core programming concepts and equip you to write programs to solve complex problems. In addition, you will gain the foundational skills a software engineer needs to solve real-world problems, from designing algorithms to testing and debugging your programs.
-----------------------------------------------------------------------------------------------------------------------------
Learn to code in Java and improve your programming and problem-solving skills. You will learn to design algorithms as well as develop and debug programs. Using custom open-source classes, you will write programs that access and transform images, websites, and other types of data. At the end of the course you will build a program that determines the popularity of different baby names in the US over time by analyzing comma separated value (CSV) files.
Build on the software engineering skills you learned in “Java Programming: Solving Problems with Software” by learning new data structures. Use these data structures to build more complex programs that use Java’s object-oriented features. At the end of the course you will write an encryption program and a program to break your encryption algorithm.
-----------------------------------------------------------------------------------------------------------------------------
Course Objectives
After completing this course you will be able to:
1. Edit, compile, and run a Java program
2. Use conditionals and loops in a Java program
3. Use Java API documentation in writing programs
4. Debug a Java program using the scientific method
5. Write a Java method to solve a specific problem
6. Develop a set of test cases as part of developing a program
7. Create a class with multiple methods that work together to solve a problem
8. Use divide-and-conquer design techniques for a program that uses multiple methods.
9. Read and write data from/to files
10. Solve problems involving data files
11. Perform quantitative analyses of data (e.g., finding maximums, minimums, averages)
12. Store and manipulate data in an array or ArrayList
13. Combine multiple classes to solve larger problems
14. Use iterables and collections (including maps) in Java.
-----------------------------------------------------------------------------------------------------------------------------
Java Fundamentals: The Java Language
-----------------------------------------------------------------------------------------------------------------------------
----------------------------------------------------------------------------------------------------
Java Interview Questions
----------------------------------------------------------------------------------------------------
1) What is Java?
Java is the high-level, object-oriented, robust, secure programming language, platform-independent, high performance, Multithreaded, and portable programming language. It was developed by James Gosling in June 1991. It can also be known as the platform as it provides its own JRE and API.
2) What do you understand by Java virtual machine?
Java Virtual Machine is a virtual machine that enables the computer to run the Java program. JVM acts like a run-time engine which calls the main method present in the Java code. JVM is the specification which must be implemented in the computer system. The Java code is compiled by JVM to be a Bytecode which is machine independent and close to the native code.
3) What are the main differences between the Java platform and other platforms?
There are the following differences between the Java platform and other platforms.
- Java is the software-based platform whereas other platforms may be the hardware platforms or software-based platforms.
- Java is executed on the top of other hardware platforms whereas other platforms can only have the hardware components.
4) What is the default value of the local variables?
The local variables are not initialized to any default value, neither primitives nor object references.
5) What are the various access specifiers in Java?
In Java, access specifiers are the keywords which are used to define the access scope of the method, class, or a variable. In Java, there are four access specifiers given below:
- Public The classes, methods, or variables which are defined as public, can be accessed by any class or method.
- Protected Protected can be accessed by the class of the same package, or by the sub-class of this class, or within the same class.
- Default Default are accessible within the package only. By default, all the classes, methods, and variables are of default scope.
- Private The private class, methods, or variables defined as private can be accessed within the class only.
6) What are the advantages of Packages in Java?
There are various advantages of defining packages in Java.
- Packages avoid the name clashes.
- The Package provides easier access control.
- We can also have the hidden classes that are not visible outside and used by the package.
- It is easier to locate the related classes.
7) What is an object?
The Object is the real-time entity having some state and behavior. In Java, Object is an instance of the class having the instance variables as the state of the object and the methods as the behavior of the object. The object of a class can be created by using the new keyword.
8) What is "this" keyword in java?
Within an instance method or a constructor, this is a reference to the current object — the object whose method or constructor is being called. You can refer to any member of the current object from within an instance method or a constructor by using this.
9) What is the constructor?
In Java, a constructor is a block of codes similar to the method. It is called when an instance of the class is created. At the time of calling constructor, memory for the object is allocated in the memory.
10) Can we override private methods in Java?
No, a private method cannot be overridden since it is not visible from any other class.
11) What is blank final variable?
A final variable in Java can be assigned a value only once, we can assign a value either in declaration or later.
12) What is "super" keyword in java?
The super keyword in java is a reference variable that is used to refer parent class objects. The keyword “super” came into the picture with the concept of Inheritance. Whenever you create the instance of a subclass, an instance of parent class is created implicitly i.e. referred by super reference variable.
13) What is static variable in Java?
The static keyword in java is used for memory management mainly. We can apply java static keyword with variables, methods, blocks and nested class. The static keyword belongs to the class than the instance of the class.
14) Differences between HashMap and HashTable in Java.
o HashMap is non synchronized. It is not-thread safe and can’t be shared between many threads without proper synchronization code whereas Hashtable is synchronized. It is thread-safe and can be shared with many threads.
o HashMap allows one null key and multiple null values whereas Hashtable doesn’t allow any null key or value.
o HashMap is generally preferred over HashTable if thread synchronization is not needed.
15) How are Java objects stored in memory?
In Java, all objects are dynamically allocated on Heap. This is different from C++ where objects can be allocated memory either on Stack or on Heap. In C++, when we allocate abject using new(), the object is allocated on Heap, otherwise on Stack if not global or static.
In Java, when we only declare a variable of a class type, only a reference is created (memory is not allocated for the object). To allocate memory to an object, we must use new(). So the object is always allocated memory on the heap.
16) What is the static method?
o A static method belongs to the class rather than the object.
o There is no need to create the object to call the static methods.
o A static method can access and change the value of the static variable.
17) What are the restrictions that are applied to the Java static methods?
Two main restrictions are applied to the static methods.
o The static method can not use non-static data member or call the non-static method directly.
o this and super cannot be used in static context as they are non-static.
18) Why is the main method static?
Because the object is not required to call the static method. If we make the main method non-static, JVM will have to create its object first and then call main() method which will lead to the extra memory allocation.
19) Can we override the static methods?
No, we can't override static methods.
20) What is the static block?
Static block is used to initialize the static data member. It is executed before the main method, at the time of classloading.
21) Explain Typecasting
The concept of assigning a variable of one data type to a variable of another data type. It is not possible for the boolean data type.
22) Explain different types of typecasting?
Different types of typecasting are:
• Implicit: Storing values from a smaller data type to the larger data type. It is automatically done by the compiler.
• Explicit: Storing the value of a larger data type into a smaller data type. This results in information loss.
23) Define classes in Java
A class is a collection of objects of similar data types. Classes are user-defined data types and behave like built-in types of a programming language.
24) What do you mean by Overloading?
Overloading is the phenomenon when two or more different methods (method overloading) or operators (operator overloading) have the same representation. For example, the + operator adds two integer values but concatenates two strings. Similarly, an overloaded function called Add can be used for two purposes:
1. To add two integers
2. To concatenate two strings
25) Could you draw a comparison between Array and ArrayList?
An array necessitates for giving the size during the time of declaration, while an array list doesn't necessarily require size as it changes size dynamically. To put an object into an array, there is the need to specify the index. However, no such requirement is in place for an array list. While an array list is parameterized, an array is not parameterized.
26) Please explain the difference between String, String Builder, and String Buffer.
String variables are stored in a constant string pool. With the change in the string reference, it becomes impossible to delete the old value. For example, if a string has stored a value "Old," then adding the new value "New" will not delete the old value. It will still be there, however, in a dormant state. In a String Buffer, values are stored in a stack. With the change in the string reference, the new value replaces the older value. The String Buffer is synchronized (and therefore, thread-safe) and offers slower performance than the String Builder, which is also a String Buffer but is not synchronized. Hence, performance is fast in String Builder than the String Buffer.
27) What is String Pool in Java?
The collection of strings stored in the heap memory refers to the String pool. Whenever a new object is created, it is checked if it is already present in the String pool or not. If it is already present, then the same reference is returned to the variable else new object is created in the String pool, and the respective reference is returned.
28) What do you know about Interface in Java?
A Java interface is a template that has only method declarations and not method implementations. It is a workaround for achieving multiple inheritances in Java.
29) How is an Abstract class different from an Interface?
There are several differences between an Abstract class and an Interface in Java, summed up as follows:
• Constituents – An abstract class contains instance variables, whereas an interface can contain only constants.
• Constructor and Instantiation – While an interface has neither a constructor nor it can be instantiated, an abstract class can have a default constructor that is called whenever the concrete subclass is instantiated.
• Implementation of Methods – All classes that implement the interface need to provide an implementation for all the methods contained by it. A class that extends the abstract class, however, doesn't require implementing all the methods contained in it. Only abstract methods need to be implemented in the concrete subclass.
• Type of Methods – Any abstract class has both abstract as well as non-abstract methods. Interface, on the other hand, has only a single abstract method.
30) Please explain what do you mean by an Abstract class and an Abstract method?
An abstract class in Java is a class that can't be instantiated. Such a class is typically used for providing a base for subclasses to extend as well as implementing the abstract methods and overriding or using the implemented methods defined in the abstract class. To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.
31) What is multiple inheritance? Does Java support multiple inheritance? If not, how can it be achieved?
If a subclass or child class has two parent classes, that means it inherits the properties from two base classes, it is multiple inheritances. Java does not multiple inheritances as in case if the parent classes have the same method names, then at runtime, it becomes ambiguous, and the compiler is unable to decide which method to execute from the child class.
32) How can we restrict inheritance for a class?
We can restrict inheritance for a class by the following steps:
1. By using the final keyword
2. If we make all methods final, then we cannot override that
3. By using private constructors
4. By using the Javadoc comment (//)
33) Are constructors inherited? Can a subclass call the parent's class constructor?
We cannot inherit a constructor. We create an instance of a subclass using a constructor of one of its superclasses. Because overriding the superclass constructor is not our wish as if we override a superclass constructor, then we will destroy the encapsulation abilities of the language.
34) Define JSON
The expansion of JSON is ‘JavaScript Object Notation.’ It is a much lighter and readable alternative to XML. It is independent and easily parse-able in all programming languages. It is primarily used for client–server and server–server communication.
35) What are the advantages of JSON over XML?
The advantages of JSON over XML are:
1. JSON is lighter and faster than XML.
2. It is easily understandable.
3. It is easy to parse and convert to objects for information consumption.
4. JSON supports multiple data types—string, number, array, or Boolean—but XML data are all strings.
36) Can we import the same package/class twice? Will the JVM load the package twice at runtime?
A package or class can be inherited multiple times in a program code. JVM and compiler will not create any issue. Moreover, JVM automatically loads the class internally once, regardless of times it is called in the program.
37) Describe annotations.
• Java annotation is a tag that symbolizes the metadata associated with class, interface, methods, fields, etc.
• Annotations do not directly influence operations.
• The additional information carried by annotations is utilized by Java compiler and JVM.
38) Java doesn't use pointers. Why?
Pointers are susceptible and slightly carelessness in their use which may result in memory problems, and hence Java basically manages their use.
39) Distinguish between static loading and dynamic class loading.
o Static loading: In static loading, classes are loaded statically with the operator ‘new.’
o Dynamic class loading: It is a technique for programmatically invoking the functions of a class loader at runtime.
40) Define an enumeration?
Usually, we call enumeration as an enum. An enumeration is an interface containing methods for accessing the original data structure from which the enumeration is obtained. It allows sequential access to all the elements stored in the collection.
----------------------------------------------------------------------------------------------------------------------------